Understanding Class Types
When individual elements or groups of elements need to diverge from the element-wide style, attaching a class allows us to make an exception. But with great power comes great responsibility, as classes can very quickly become a messy spider web of interdependencies and over-specification, which is why a utility class library can keep things orderly. But even using the most robust utility class library, many projects will still require creating new custom classes. And one thing to consider when creating these classes is that adjustments may need to be made in the future, which makes it important to author classes in ways that don’t need to be untangled at a later date.
To understand how to create and maintain a usable class library, it's important to dig into the different types of classes and how they relate to one another.
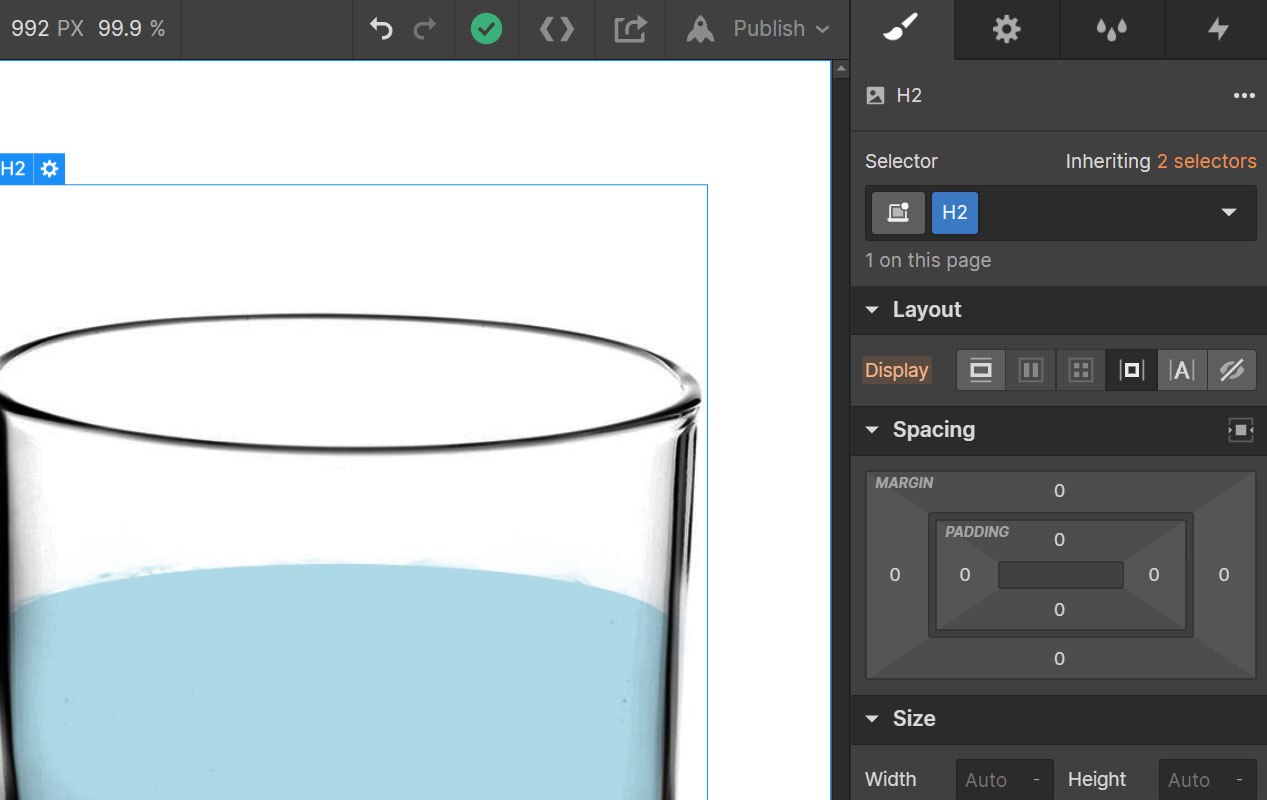
Utility Classes
A utility class can be thought of like a chemical element, such as <class>H2<class> (Hydrogen).
<class>H2<class>
A utility class, on its own, represents a very specific behavior. <class>H2<class>, for instance, might represent a blue background. And <class>O<class> (Oxygen) might represent a bubble background image. On its own, the usefulness of a utility class can be limited, but they can be combined with other classes to achieve more sophisticated results.
Combining Utility Classes
Utility classes can be strung together to behave as the sum of their parts. Using the above example, if we combine <class>H2<class> and <class>O<class>, we'll end up with the bubble background image on top of a blue background, combining to represent the appearance of water.
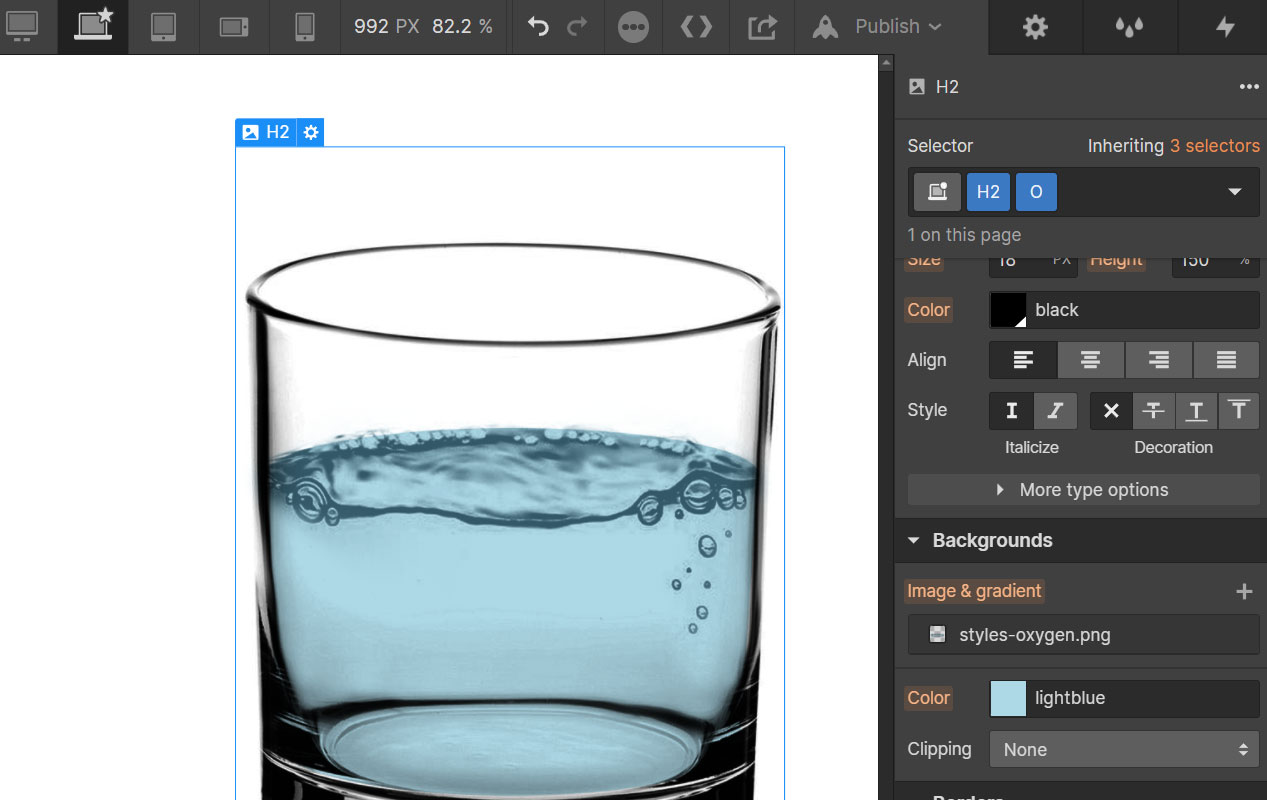
<class>H2<class> <class>O<class>
Using utility classes like a library of elements, we can string them together to quickly construct most common styles found on the web without having to name, declare or maintain complex classes.
When simple classes are compounded together, declaring styles thereafter will create a Combo Class (discussed below) and the styles will be dependent on all applied simple classes being present. So when creating or editing simple classes that are a part of a compounded list of classes, it's essential to create a new "dummy" div or text element, apply the class, & make declarations directly to the simple class.
Custom Classes
Custom classes are created for a specific situation and may have a name that doesn't necessarily describe its make-up or behavior. For instance, we might create a class that has all of the properties of <class>H2<class> <class>O<class>, but name it <class>Water<class>.
<class>Water<class>
These are useful to achieve behaviors that aren't easily supported by simple utility classes, but the drawback is that their behavior might not be obvious from the class name alone. We might have to refer to the style panel to see exactly what CSS properties were declared.
Combo Classes
Combo classes behave a little like chemical reactions in that when two particular classes are combined (in any order), the resulting behavior is more than the sum of its parts. For instance, if we drop <class>K<class> (Potassium) in a glass of water, the unexpected reaction will be a flame...
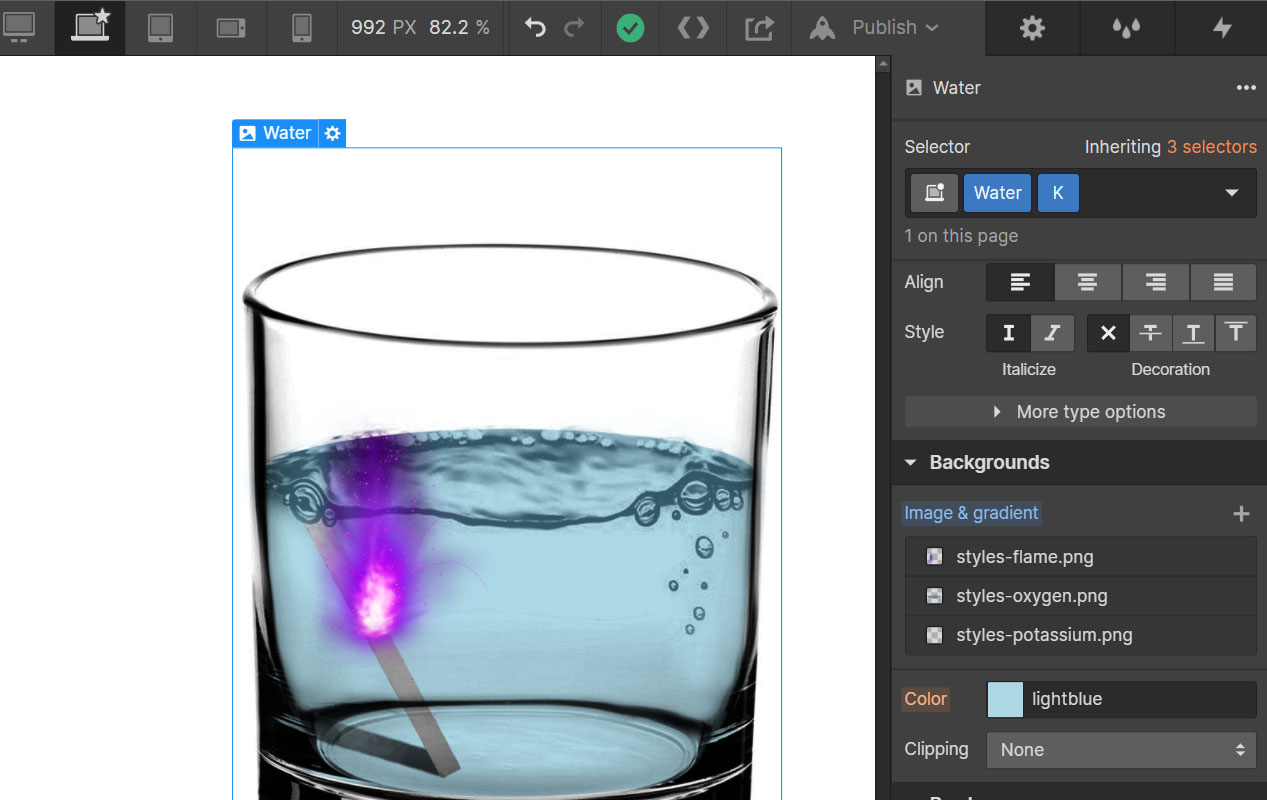
<class>Water<class> <class>K<class>
In other words, the whole is somehow unique to the sum of the individual parts. The flame only shows up when both the class <class>Water<class> and <class>K<class> are present. This is the case any time you have multiple classes added to an element and you see styles applied with a blue header. And in order to edit this combo class in the future, you'll need to recreate the string of classes in the exact same order.